PlaceSearch.js
Create <input>
fields with search ahead address autocomplete
Getting Started
To get started, first include PlaceSearch.js and PlaceSearch.css in your HTML header.
<script src="https://api.mqcdn.com/sdk/place-search-js/v1.0.0/place-search.js"></script>
<link type="text/css" rel="stylesheet" href="https://api.mqcdn.com/sdk/place-search-js/v1.0.0/place-search.css"/>
Setup the <input>
element you want to use.
<input type="search" id="place-search-input" placeholder="Start Searching..."/>
Then initialize your MapQuest key and the reference to the input field element.
placeSearch({
key: 'KEY',
container: document.querySelector('#place-search-input')
});
Options
In the list below are the options you can pass to the placeSearch(options)
function:
-
key required
The API Key, which is needed to make requests to MapQuest services.
-
container required
Determines the
input
that will be used for the dropdown menu.Pass a reference to an Element. Obtained via document.querySelector for example.
-
collection
Search Ahead organizes types of data into collections, which can be requested using this parameter.
Franchise and category collections vary and return category (SIC) codes to be used with the Place Search API
Available Collections
- address
- adminArea
- airport
- category
- franchise
- poi
Default:
['airport', 'address', 'adminArea', 'poi']
-
limit
Determines the number of results returned from the Search Ahead API.
Default: 5. Must lie in the range [1, 15].
-
style
Control whether the default styles should be used.
Default: Styles are enabled,
true
. -
templates
Change the templates used.
Available templates:
- header: Rendered above the results list.
- value
- suggestion
- footer: Rendered below the results list.
- empty: If no results are returned, this is rendered.
Each template is a function that will receive a result object and must return a string.
Value must return a plain string as it's used to fill the input.value. suggestion should return an HTML string to be displayed in the dropdown.
Header, footer, and empty should return an HTML string.
-
autocompleteOptions
The PlaceSearch.js library is leveraging Algolia's autocomplete.js
autocomplete.js options to configure the underlying autocomplete.js instance.
-
useDeviceLocation
Ask and use the device location.
Default:
false
.
Methods
The PlaceSearch.js library provides a few methods to interact with:
-
open
Opens the dropdown menu. The menu will be visible if it has content to show.
Example
placeSearch.open()
-
close
Closes the dropdown menu.Example
placeSearch.close()
-
getVal
Get the current input value.
Example
placeSearch.getVal()
-
setVal
Events
-
change Event
This event is fired when a result is selected in the dropdown.
Example
placeSearch.on('change', (e) => { console.log(e); });
-
results Event
This event is fired when the dropdown receives results. You will receive the array of results that are displayed.
This event is also fired when no results are found.
Example
placeSearch.on('results', (e) => { console.log(e); });
-
cursorchanged Event
This event is fired when arrows keys are used to navigate suggestions.
Example
placeSearch.on('cursorchanged', (e) => { console.log(e); });
-
clear Event
This event is fired when the PlaceSearch input is cleared. This event doesn't return anything, as the value of the query is then
''
and no results are displayed.Example
placeSearch.on('clear', (e) => { console.log(e); });
-
error Event
This event is fired when the request to the MapQuest Search Ahead servers fails for any reason.
Example
placeSearch.on('clear', (e) => { console.log(e); });
Result Object
Events will return result objects with the properties below:
Key | Description |
---|---|
type
|
A string that contains information about the type of result that is returned. Possible
values are: |
name
|
A string that contains the name of the result that is returned without additional summary information included. |
city
|
A string that contains the enclosing city of this location, if applicable to the result returned. |
state
|
A string that contains the enclosing state or province of this location, if applicable to the result returned. |
stateCode
|
A string that contains the enclosing two-character state code of this location, if applicable to the result returned. |
country
|
A string that contains the enclosing country of this location, if applicable to the result returned. |
countryCode
|
A string that contains the enclosing ISO 3166-1 Alpha-2 country code of this location, if applicable to the result returned. |
latLng
|
An object that contains the longitude and latitude that describes the geographic location of the result that is returned. |
postalCode
|
A string that contains the coarsest enclosing postal code, if applicable to the result returned. |
searchAheadResult
|
The result object returned by the Search Ahead API. |
value
|
A string that contains a summary of the result that is returned. Depending on the type
of result returned, the |
Search Ahead API
The PlaceSearch.js library is powered by our Search Ahead API in the background.
HTML structure
Here is what the wrapper and input field html structure looks like:
<span class="mq-place-search">
<input class="mq-input" />
<button class="mq-input-icon mq-icon-clear"><svg></svg></button>
</span>
Here is what the dropdown html structure looks like:
<span class="mq-dropdown-menu mq-with-results">
<div>
<span class="mq-results">
<div class="mq-result">
<span class="mq-result-icon"><svg></svg></span>
<span class="mq-name"></span>
<span class="mq-address"></span>
</div>
<!-- More Results-->
</span>
</div>
</span>
CSS classes
Below is a visual overlay of the different CSS classes in PlaceSearch.js:
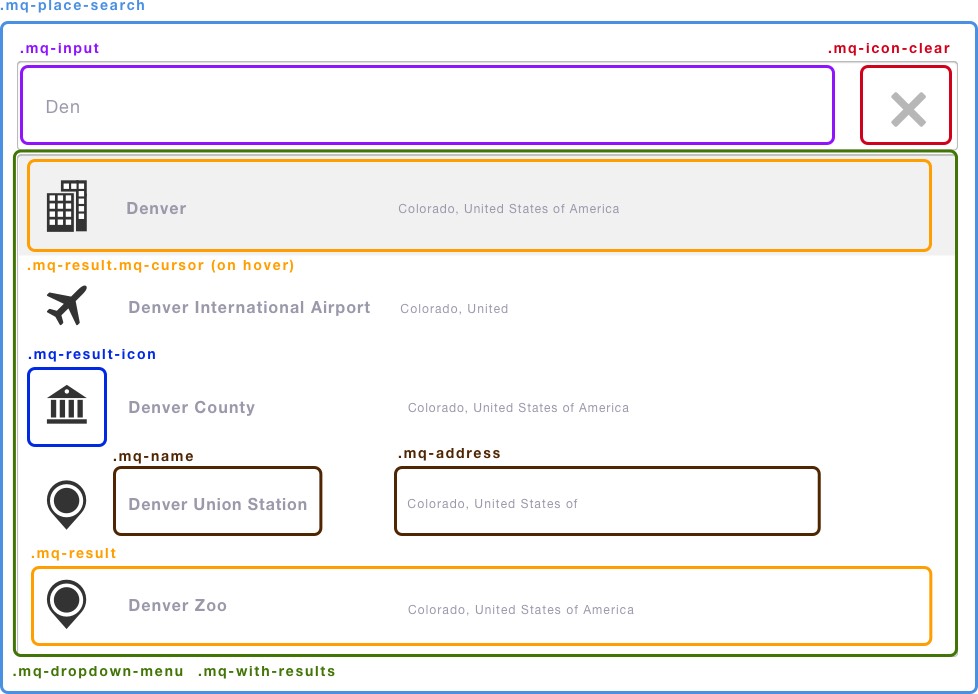
CSS Class | Description |
---|---|
This class is the one used by the main PlaceSearch input. |
|
.mq-icon-clear
|
This is the class attached to the clear icon, which is visible when the input has characters, clicking it will empty the input. |
This class is the one that is on the whole results wrapper. |
|
.mq-result
|
The .mq-result class is the class of each dropdown result. |
.mq-cursor
|
The .mq-cursor class is added to .mq-result when the result is hovered. |
.mq-result-icon
|
The .mq-result-icon is the class that is used on the left icon of each result. |
.mq-name
|
The .mq-name class is used on the name of the place. |
.mq-address
|
The .mq-address class is used on the address of the place. |
Below is blank css that can be used to customize the default styling.
.mq-place-search {}
.mq-input {}
.mq-input::-webkit-search-decoration {}
.mq-input::-webkit-search-cancel-button {}
.mq-input::-ms-clear {}
.mq-input-icon {}
.mq-input-icon svg {}
.mq-dropdown-menu {}
.mq-result {}
.mq-result .mq-name {}
.mq-result .mq-address {}
.mq-result-icon {}
.mq-result-icon svg {}
.mq-cursor {}
.mq-cursor .mq-result-icon svg {}
.mq-icon-clear {}
Disable Default Styles
When initializing PlaceSearch.js, you can disable the style/formatting of input completely. The CSS classes
names
with be prepended with mq-no-style
instead of mq-
.
placeSearch({
key: 'KEY',
container: document.querySelector('#place-search-input'),
style: false
});
License
The PlaceSearch.js library is leveraging Algolia's autocomplete.js library which is licensed under MIT.
MapQuest data is licensed, see our Terms and Conditions.
Cost
Each Search Ahead API request that is made costs 1/10th of a transaction. So for every 10 requests you make, you are charged 1 transaction according to our Plans Page. The PlaceSearch.js SDK doesn't start making requests to the Search Ahead API until the second character is typed.
Examples
Check out our examples for inspiration.